Introduction: C++ is a powerful and versatile programming language widely used in various domains, including software development, game development, system programming, and more. Whether you’re just starting your programming journey or looking to deepen your understanding of C++, this comprehensive tutorial will guide you through the fundamentals and advanced concepts of the language. From basic syntax to advanced topics like templates and multithreading, this tutorial aims to equip you with the knowledge and skills needed to become proficient in C++ programming.
Part 1: Beginner Level
- Introduction to C++:
- Overview of C++ and its features.
- History and evolution of the language.
- Setting up the development environment.
- Getting Started:
- Writing your first “Hello, World!” program.
- Understanding basic syntax: variables, data types, and operators.
- Input and output using cin and cout.
- Control Flow:
- Conditional statements: if, else-if, and switch.
- Looping constructs: for, while, and do-while.
- Break and continue statements.
- Functions and Scope:
- Declaring and defining functions.
- Function parameters and return types.
- Scope rules and variable visibility.
- Arrays and Pointers:
- Declaring and accessing arrays.
- Pointer basics: memory addresses and dereferencing.
- Pointer arithmetic and dynamic memory allocation.
- Object-Oriented Programming (OOP) Basics:
- Classes and objects.
- Encapsulation, inheritance, and polymorphism.
- Constructors and destructors.
- Exception Handling:
- Handling errors and exceptions using try-catch blocks.
- Throwing and catching exceptions.
- File Handling:
- Reading from and writing to files.
- File streams and file manipulation.
Part 2: Advanced Level
- Templates:
- Generic programming with templates.
- Function templates and class templates.
- Template specialization and template metaprogramming.
- Standard Template Library (STL):
- Overview of STL containers: vectors, lists, maps, etc.
- Algorithms: sorting, searching, and manipulating containers.
- Iterators and iterator categories.
- Smart Pointers:
- Introduction to smart pointers: unique_ptr, shared_ptr, and weak_ptr.
- Memory management and automatic resource cleanup.
- Lambda Expressions:
- Writing inline anonymous functions using lambda expressions.
- Capturing variables by value and reference.
- Multithreading:
- Creating and managing threads.
- Synchronization primitives: mutexes, condition variables, and atomic operations.
- Thread safety and avoiding race conditions.
- Move Semantics and Rvalue References:
- Understanding move semantics and the move constructor.
- Rvalue references and perfect forwarding.
- Advanced Topics:
- RAII (Resource Acquisition Is Initialization) idiom.
- Type deduction: auto keyword and decltype specifier.
- User-defined literals and constexpr functions.
Conclusion: By following this comprehensive tutorial, you’ve embarked on a journey to master the C++ programming language, starting from the fundamentals and progressing to advanced topics. Remember, practice is key to becoming proficient in C++, so be sure to apply what you’ve learned through coding exercises and projects. With dedication and perseverance, you’ll soon be able to leverage the full power and flexibility of C++ to build robust and efficient software solutions. Happy coding!
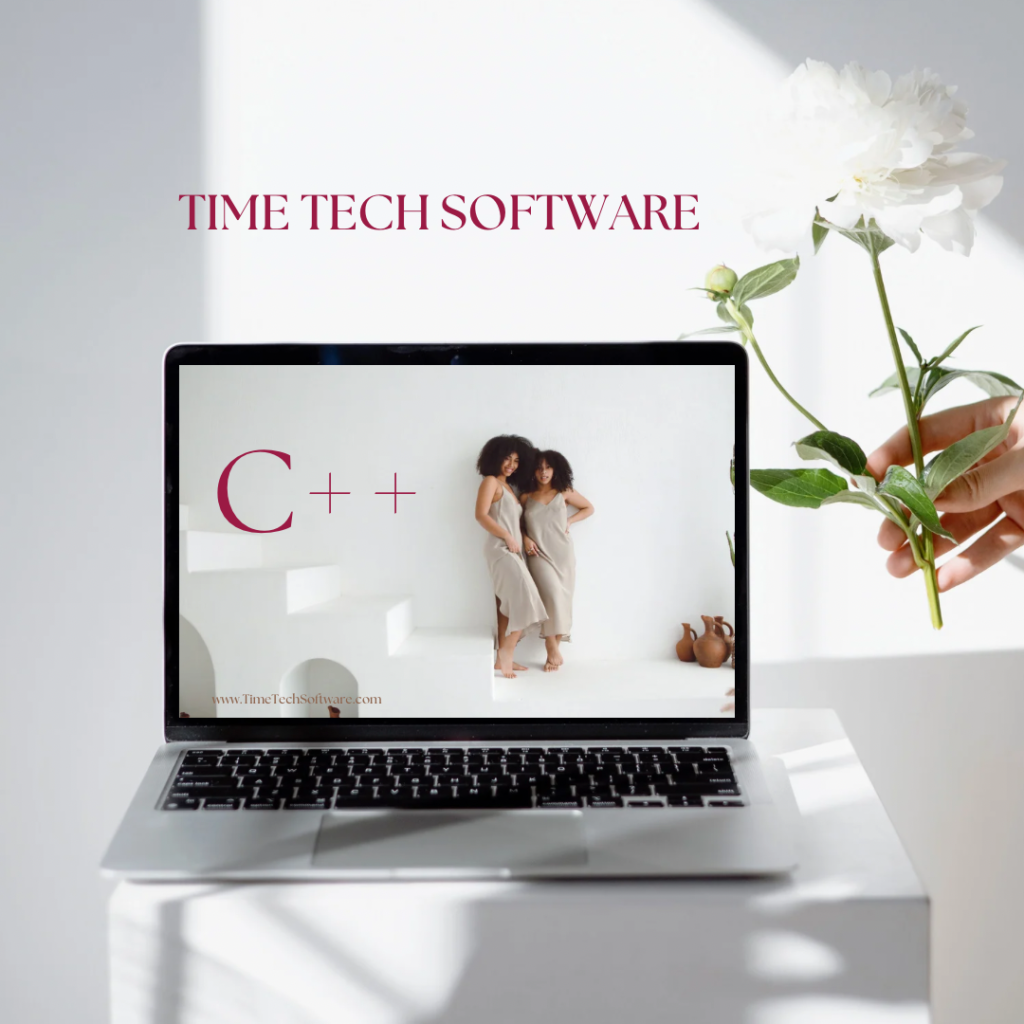